mirror of
https://github.com/filebrowser/filebrowser.git
synced 2025-05-11 20:53:00 +00:00
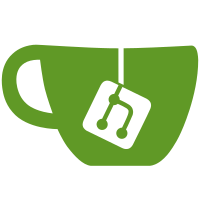
* Update dependencies and remove typescript version pinning (fixed upstream) * Fix esling warnings (disabled any and script lang checks) Rewrote clipboard copy (Fixes #3407) Run prettier --------- Co-authored-by: Oleg Lobanov <oleg@lobanov.me>
84 lines
1.6 KiB
TypeScript
84 lines
1.6 KiB
TypeScript
function loading(button: string) {
|
|
const el: HTMLButtonElement | null = document.querySelector(
|
|
`#${button}-button > i`
|
|
);
|
|
|
|
if (el === undefined || el === null) {
|
|
console.log("Error getting button " + button);
|
|
return;
|
|
}
|
|
|
|
if (el.innerHTML == "autorenew" || el.innerHTML == "done") {
|
|
return;
|
|
}
|
|
|
|
el.dataset.icon = el.innerHTML;
|
|
el.style.opacity = "0";
|
|
|
|
setTimeout(() => {
|
|
if (el) {
|
|
el.classList.add("spin");
|
|
el.innerHTML = "autorenew";
|
|
el.style.opacity = "1";
|
|
}
|
|
}, 100);
|
|
}
|
|
|
|
function done(button: string) {
|
|
const el: HTMLButtonElement | null = document.querySelector(
|
|
`#${button}-button > i`
|
|
);
|
|
|
|
if (el === undefined || el === null) {
|
|
console.log("Error getting button " + button);
|
|
return;
|
|
}
|
|
|
|
el.style.opacity = "0";
|
|
|
|
setTimeout(() => {
|
|
if (el !== null) {
|
|
el.classList.remove("spin");
|
|
el.innerHTML = el?.dataset?.icon || "";
|
|
el.style.opacity = "1";
|
|
}
|
|
}, 100);
|
|
}
|
|
|
|
function success(button: string) {
|
|
const el: HTMLButtonElement | null = document.querySelector(
|
|
`#${button}-button > i`
|
|
);
|
|
|
|
if (el === undefined || el === null) {
|
|
console.log("Error getting button " + button);
|
|
return;
|
|
}
|
|
|
|
el.style.opacity = "0";
|
|
|
|
setTimeout(() => {
|
|
if (el !== null) {
|
|
el.classList.remove("spin");
|
|
el.innerHTML = "done";
|
|
el.style.opacity = "1";
|
|
}
|
|
setTimeout(() => {
|
|
if (el) el.style.opacity = "0";
|
|
|
|
setTimeout(() => {
|
|
if (el !== null) {
|
|
el.innerHTML = el?.dataset?.icon || "";
|
|
el.style.opacity = "1";
|
|
}
|
|
}, 100);
|
|
}, 500);
|
|
}, 100);
|
|
}
|
|
|
|
export default {
|
|
loading,
|
|
done,
|
|
success,
|
|
};
|