mirror of
https://github.com/filebrowser/filebrowser.git
synced 2025-06-30 21:22:58 +00:00
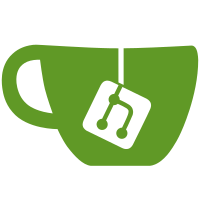
--------- Co-authored-by: Joep <jcbuhre@gmail.com> Co-authored-by: Omar Hussein <omarmohammad1951@gmail.com> Co-authored-by: Oleg Lobanov <oleg@lobanov.me>
46 lines
1023 B
TypeScript
46 lines
1023 B
TypeScript
import { fetchURL, fetchJSON, removePrefix, createURL } from "./utils";
|
|
|
|
export async function list() {
|
|
return fetchJSON<Share[]>("/api/shares");
|
|
}
|
|
|
|
export async function get(url: string) {
|
|
url = removePrefix(url);
|
|
return fetchJSON<Share>(`/api/share${url}`);
|
|
}
|
|
|
|
export async function remove(hash: string) {
|
|
await fetchURL(`/api/share/${hash}`, {
|
|
method: "DELETE",
|
|
});
|
|
}
|
|
|
|
export async function create(
|
|
url: string,
|
|
password = "",
|
|
expires = "",
|
|
unit = "hours"
|
|
) {
|
|
url = removePrefix(url);
|
|
url = `/api/share${url}`;
|
|
if (expires !== "") {
|
|
url += `?expires=${expires}&unit=${unit}`;
|
|
}
|
|
let body = "{}";
|
|
if (password != "" || expires !== "" || unit !== "hours") {
|
|
body = JSON.stringify({
|
|
password: password,
|
|
expires: expires.toString(), // backend expects string not number
|
|
unit: unit,
|
|
});
|
|
}
|
|
return fetchJSON(url, {
|
|
method: "POST",
|
|
body: body,
|
|
});
|
|
}
|
|
|
|
export function getShareURL(share: Share) {
|
|
return createURL("share/" + share.hash, {}, false);
|
|
}
|