mirror of
https://github.com/filebrowser/filebrowser.git
synced 2025-05-08 11:22:10 +00:00
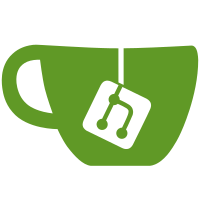
* Change the order of commands to be able to cache more layers in case of multiple builds triggered in a row * Fix #471 * Format Code * Revert "Change the order of commands to be able to cache more layers in case of multiple builds triggered in a row" This reverts commit 29217f66ee6aee63d2c03ac86de4ad437876317d [formerly ebff3e9d79ac9eca44d7b3caf7814be62c784d43] [formerly 9b95d9e986254d55405cd0e9484dcbbadc54c87b [formerly d13fd2878c38a46f91da30de150624200f0b32e9]] [formerly 3ec8fb12d8b6e1942ebae6abb00c5f15b03d6412 [formerly 6a70bdaf457f50896dd9826608666a39babae666] [formerly 063a6fe9d4991b7b6c257ae081288ea40efbe8b5 [formerly 01362f34ee45b342f4e9148730ccd30027e5aebf]]]. * Adjustment based on the review * Rename "login-header" to "loginHeader" and prepare auth.method to accept "none" as a value * Fixed line break * Readd "lumberjack.v2" import which was removed by gofmt Sorry - I do my tests and run "gofmt" before comitting the changes - It sadly seems like it is messing up the imports over and over again. Former-commit-id: 252e65171f70ee87238b5542e6af81d90bdaed6b [formerly fa843827feaab389550f32ba3a629e1968bcea3d] [formerly 942986226dbb56ef1cb4dff24445406cfa699d2d [formerly ed62451ea0c19d7d0ec25b0290cce4819e2dc514]] Former-commit-id: e87377dd6f30012b0d602b592100a7deb39a8632 [formerly f8198aa8a51fd5e727c31df0918ab62024520cef] Former-commit-id: 019de07d53c3da16354e228330c14efb0dfb2122
78 lines
2.4 KiB
Go
78 lines
2.4 KiB
Go
/*
|
|
Package filebrowser provides a web interface to access your files
|
|
wherever you are. To use this package as a middleware for your app,
|
|
you'll need to import both File Browser and File Browser HTTP packages.
|
|
|
|
import (
|
|
fm "github.com/filebrowser/filebrowser"
|
|
h "github.com/filebrowser/filebrowser/http"
|
|
)
|
|
|
|
Then, you should create a new FileBrowser object with your options. In this
|
|
case, I'm using BoltDB (via Storm package) as a Store. So, you'll also need
|
|
to import "github.com/filebrowser/filebrowser/bolt".
|
|
|
|
db, _ := storm.Open("bolt.db")
|
|
|
|
m := &fm.FileBrowser{
|
|
NoAuth: false,
|
|
Auth: {
|
|
Method: "default",
|
|
LoginHeader: "X-Fowarded-User"
|
|
},
|
|
DefaultUser: &fm.User{
|
|
AllowCommands: true,
|
|
AllowEdit: true,
|
|
AllowNew: true,
|
|
AllowPublish: true,
|
|
Commands: []string{"git"},
|
|
Rules: []*fm.Rule{},
|
|
Locale: "en",
|
|
CSS: "",
|
|
Scope: ".",
|
|
FileSystem: fileutils.Dir("."),
|
|
},
|
|
Store: &fm.Store{
|
|
Config: bolt.ConfigStore{DB: db},
|
|
Users: bolt.UsersStore{DB: db},
|
|
Share: bolt.ShareStore{DB: db},
|
|
},
|
|
NewFS: func(scope string) fm.FileSystem {
|
|
return fileutils.Dir(scope)
|
|
},
|
|
}
|
|
|
|
The credentials for the first user are always 'admin' for both the user and
|
|
the password, and they can be changed later through the settings. The first
|
|
user is always an Admin and has all of the permissions set to 'true'.
|
|
|
|
Then, you should set the Prefix URL and the Base URL, using the following
|
|
functions:
|
|
|
|
m.SetBaseURL("/")
|
|
m.SetPrefixURL("/")
|
|
|
|
The Prefix URL is a part of the path that is already stripped from the
|
|
r.URL.Path variable before the request arrives to File Browser's handler.
|
|
This is a function that will rarely be used. You can see one example on Caddy
|
|
filemanager plugin.
|
|
|
|
The Base URL is the URL path where you want File Browser to be available in. If
|
|
you want to be available at the root path, you should call:
|
|
|
|
m.SetBaseURL("/")
|
|
|
|
But if you want to access it at '/admin', you would call:
|
|
|
|
m.SetBaseURL("/admin")
|
|
|
|
Now, that you already have a File Browser instance created, you just need to
|
|
add it to your handlers using m.ServeHTTP which is compatible to http.Handler.
|
|
We also have a m.ServeWithErrorsHTTP that returns the status code and an error.
|
|
|
|
One simple implementation for this, at port 80, in the root of the domain, would be:
|
|
|
|
http.ListenAndServe(":80", h.Handler(m))
|
|
*/
|
|
package filebrowser
|