mirror of
https://github.com/filebrowser/filebrowser.git
synced 2025-05-08 19:22:57 +00:00
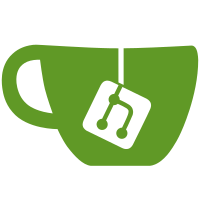
Former-commit-id: 99d241dc790629791b67b7b2ce20a10341a1c1e7 [formerly 08e8410bffdb50134a2d6ccbe853b25d4c4d3fc6] [formerly f32b18d9acb42ee1988c49b7980a16a606cee142 [formerly 1080bfde680d9d87271e63e6253450595e1f5c05]] Former-commit-id: 318c4f159697e896bcf824e274907c5c03046bf0 [formerly 82533ae817673dfab8d56533f15a9931c0c74c1e] Former-commit-id: ece42aa8e8c7480a5a21614b6ca397b71a01e1fa
79 lines
2.2 KiB
Go
79 lines
2.2 KiB
Go
package cmd
|
|
|
|
import (
|
|
"errors"
|
|
"fmt"
|
|
"os"
|
|
"strings"
|
|
|
|
"github.com/asdine/storm"
|
|
"github.com/filebrowser/filebrowser/v2/settings"
|
|
"github.com/spf13/cobra"
|
|
v "github.com/spf13/viper"
|
|
)
|
|
|
|
func init() {
|
|
configCmd.AddCommand(configInitCmd)
|
|
rootCmd.AddCommand(configInitCmd)
|
|
addConfigFlags(configInitCmd)
|
|
configInitCmd.MarkFlagRequired("scope")
|
|
}
|
|
|
|
var configInitCmd = &cobra.Command{
|
|
Use: "init",
|
|
Short: "Initialize a new database",
|
|
Long: `Initialize a new database to use with File Browser. All of
|
|
this options can be changed in the future with the command
|
|
"filebrowser config set". The user related flags apply
|
|
to the defaults when creating new users and you don't
|
|
override the options.`,
|
|
Args: cobra.NoArgs,
|
|
Run: func(cmd *cobra.Command, args []string) {
|
|
databasePath := v.GetString("database")
|
|
if _, err := os.Stat(databasePath); err == nil {
|
|
panic(errors.New(databasePath + " already exists"))
|
|
}
|
|
|
|
defaults := settings.UserDefaults{}
|
|
getUserDefaults(cmd, &defaults, true)
|
|
authMethod, auther := getAuthentication(cmd)
|
|
|
|
db, err := storm.Open(databasePath)
|
|
checkErr(err)
|
|
defer db.Close()
|
|
st := getStorage(db)
|
|
s := &settings.Settings{
|
|
Key: generateRandomBytes(64), // 256 bit
|
|
BaseURL: mustGetString(cmd, "baseURL"),
|
|
Log: mustGetString(cmd, "log"),
|
|
Signup: mustGetBool(cmd, "signup"),
|
|
Shell: strings.Split(strings.TrimSpace(mustGetString(cmd, "shell")), " "),
|
|
AuthMethod: authMethod,
|
|
Defaults: defaults,
|
|
Server: settings.Server{
|
|
Address: mustGetString(cmd, "address"),
|
|
Port: mustGetInt(cmd, "port"),
|
|
TLSCert: mustGetString(cmd, "tls.cert"),
|
|
TLSKey: mustGetString(cmd, "tls.key"),
|
|
},
|
|
Branding: settings.Branding{
|
|
Name: mustGetString(cmd, "branding.name"),
|
|
DisableExternal: mustGetBool(cmd, "branding.disableExternal"),
|
|
Files: mustGetString(cmd, "branding.files"),
|
|
},
|
|
}
|
|
|
|
err = st.Settings.Save(s)
|
|
checkErr(err)
|
|
err = st.Auth.Save(auther)
|
|
checkErr(err)
|
|
|
|
fmt.Printf(`
|
|
Congratulations! You've set up your database to use with File Browser.
|
|
Now add your first user via 'filebrowser users new' and then you just
|
|
need to call the main command to boot up the server.
|
|
`)
|
|
printSettings(s, auther)
|
|
},
|
|
}
|